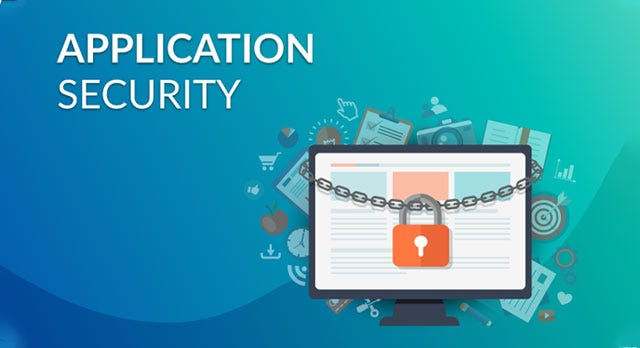
What is application security?
Application security describes security measures at the application level that aim to prevent data or code within the app from being stolen or hijacked. It encompasses the security considerations that happen during application development and design, but it also involves systems and approaches to protect apps after they get deployed.
Best Practices for Securing Containers
Application security definition
Application security is the process of developing, adding, and testing security features within applications to prevent security vulnerabilities against threats such as unauthorized access and modification.
Why application security is important
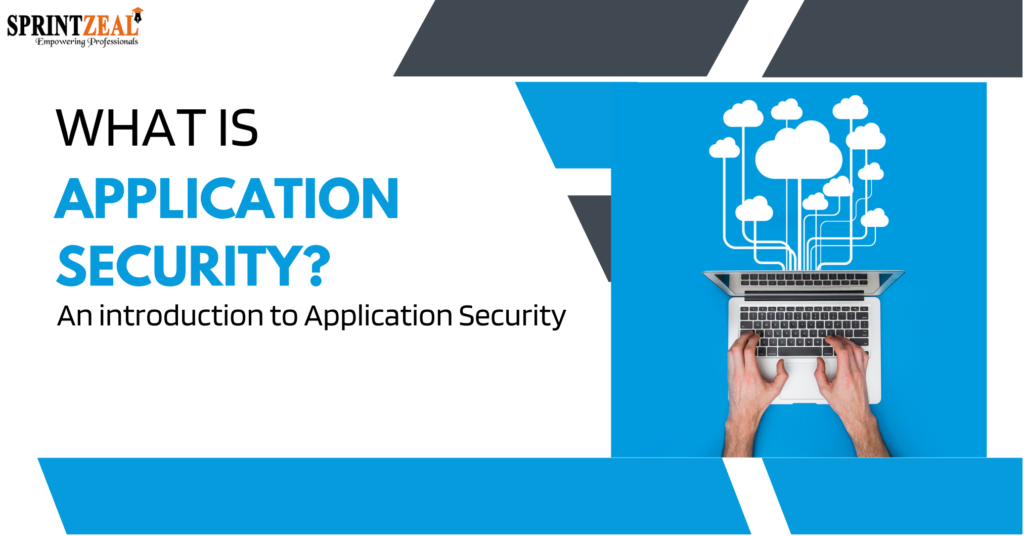
Application security is important because today’s applications are often available over various networks and connected to the cloud , increasing vulnerabilities to security threats and breaches. There is increasing pressure and incentive to not only ensure security at the network level but also within applications themselves. One reason for this is because hackers are going after apps with their attacks more today than in the past. Application security testing can reveal weaknesses at the application level, helping to prevent these attacks.
Types of application security
Introduction to Application Security
Application security refers to the process of making applications more secure by identifying, fixing, and protecting against vulnerabilities throughout the development lifecycle. With the proliferation of web and mobile applications, application security has become a critical aspect of ensuring data integrity, protecting sensitive information, and maintaining trust with users. Every software application—whether it is a cloud-based service, a web application, or a desktop program—must be designed with security in mind to prevent malicious actors from exploiting weaknesses.
The core goals of application security include:
- Confidentiality: Ensuring data is only accessible by those authorized to view it.
- Integrity: Ensuring data remains unaltered during transmission or storage.
- Availability: Ensuring that legitimate users have continuous access to data and services.
Application security is implemented through various methods and practices such as secure coding techniques, encryption, threat modeling, pen testing, and more. These are essential components of defending applications against attack vectors such as injection attacks, cross-site scripting (XSS), session hijacking, and denial-of-service (DoS) attacks.
Key Concepts in Application Security
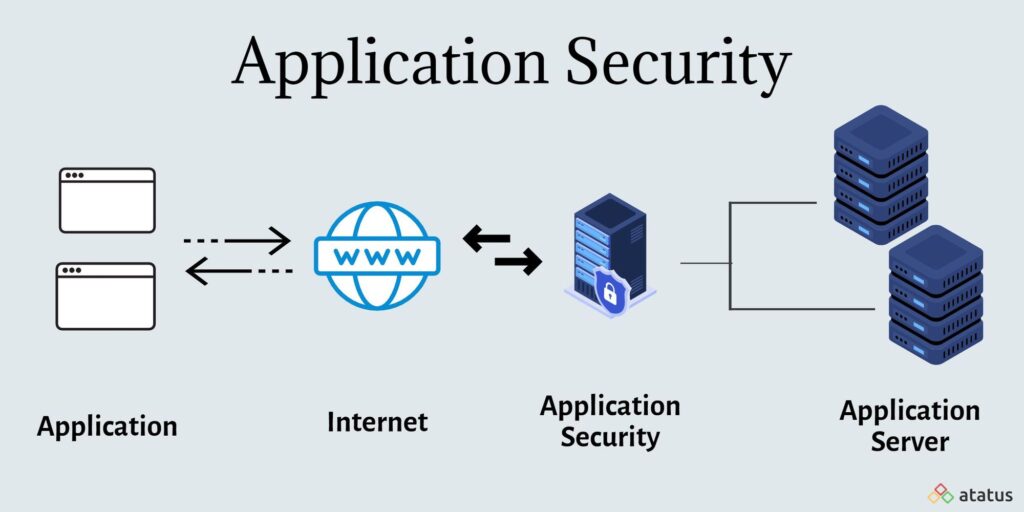
1. Authentication and Authorization
Authentication and authorization form the backbone of access control within applications.
- Authentication: Verifying the identity of a user or system. This is often done using credentials such as username/password combinations, biometrics, smart cards, or OAuth tokens. Authentication mechanisms must be robust to prevent unauthorized access through methods like password cracking or credential stuffing.
- Authorization: Once the identity is confirmed, authorization ensures that the authenticated entity can access the specific resources it is allowed to interact with. Common methods of authorization include Role-Based Access Control (RBAC) and Attribute-Based Access Control (ABAC). These ensure that users are granted permissions based on their role or attributes such as department, job level, etc.
In modern applications, Multi-Factor Authentication (MFA) adds an extra layer of security by requiring users to present multiple forms of identification before gaining access, such as a password and a one-time code from a mobile app or SMS.
2. Encryption
Encryption ensures that sensitive data, both in transit and at rest, is kept confidential and secure. There are two primary types of encryption:
- Symmetric Encryption: The same key is used for both encrypting and decrypting the data. This method is fast but requires secure key exchange between parties. AES (Advanced Encryption Standard) is a common example of symmetric encryption used for securing sensitive information.
- Asymmetric Encryption: This involves two keys: a public key and a private key. Data encrypted with a public key can only be decrypted with the corresponding private key and vice versa. This method, though more computationally expensive, allows secure communication without the need to share a secret key in advance. RSA and ECC (Elliptic Curve Cryptography) are widely used in this category.
End-to-end encryption (E2EE), especially in messaging apps, ensures that only the communicating users can read the messages, providing a layer of protection against eavesdropping.
3. Input Validation
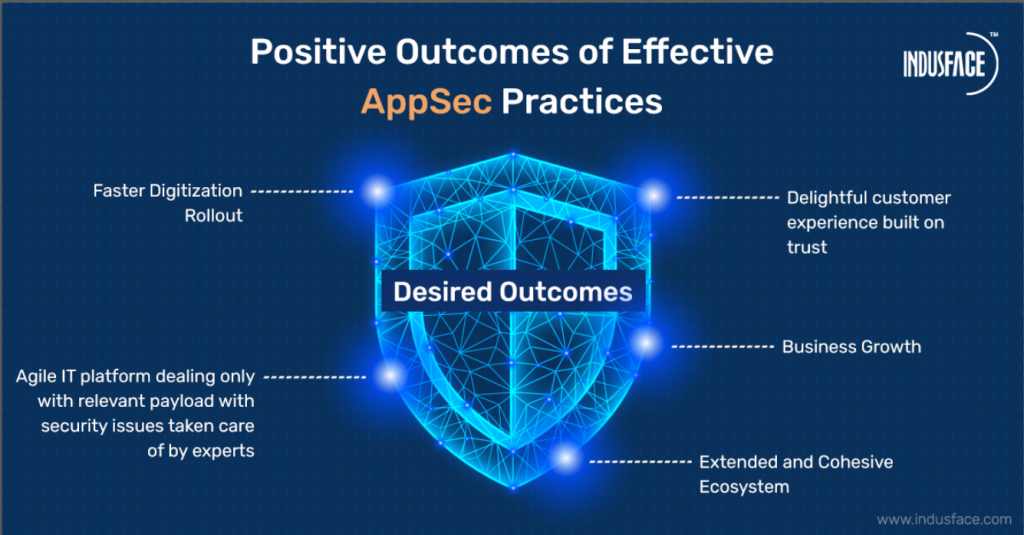
One of the most common causes of vulnerabilities in applications is improper input validation. Attackers often exploit weak input validation processes to inject malicious code into applications (such as SQL injection) or manipulate data to crash systems.
- Sanitizing Inputs: Input validation ensures that data coming from the user is checked and cleaned before being processed. This involves:
- Checking data types (e.g., numeric, string, boolean).
- Limiting the length of the input (e.g., preventing buffer overflow).
- Escaping special characters (e.g., for SQL or HTML).
- Regular Expressions: These can be used to enforce specific formatting rules for input data, such as validating email addresses or phone numbers.
Output encoding and escaping are also essential practices to ensure that untrusted data is properly handled when displayed on a web page, mitigating the risks of XSS attacks.
4. Security Headers
Security headers help to protect web applications by setting important rules for browsers when interacting with your site. These headers can mitigate attacks such as XSS, clickjacking, and content sniffing.
Key security headers include:
- Content Security Policy (CSP): CSP defines allowed sources of content on your website, helping to prevent XSS attacks by restricting the execution of scripts from unauthorized sources.
- X-Frame-Options: This header prevents your site from being embedded in an iframe, protecting against clickjacking attacks.
- X-Content-Type-Options: This ensures that browsers respect the MIME types set by your server, avoiding content-type sniffing attacks.
Secure Software Development Lifecycle (SDLC)
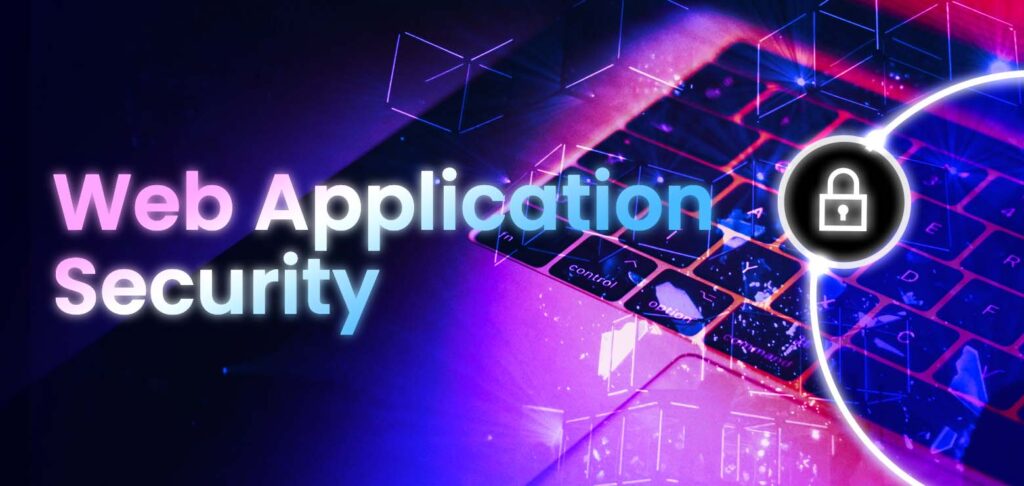
A secure SDLC is a structured approach to incorporating security into every phase of the software development process, ensuring that security risks are identified and mitigated early in development. The goal is to embed security into the foundation of the software, rather than patching vulnerabilities after release.
1. Requirements Gathering
During the requirements phase, security must be a priority. This means identifying regulatory requirements (such as GDPR or HIPAA) as well as potential threat scenarios specific to the application. Threat modeling can be used at this stage to envision how attackers might exploit weaknesses.
2. Design
The design phase should integrate security principles such as least privilege, defense in depth, and secure by design. Security architects should design the system to minimize attack surfaces, compartmentalize critical functions, and ensure secure access controls.
3. Implementation
During development, secure coding practices are crucial. Developers should be trained to avoid common security pitfalls like improper input handling, insecure cryptographic functions, and poor session management. Using automated tools like static code analyzers can help identify vulnerabilities early in the coding process.
4. Testing
Before deployment, applications must undergo rigorous security testing, including:
- Static Application Security Testing (SAST): Examining source code for vulnerabilities without executing the code.
- Dynamic Application Security Testing (DAST): Running the application in real time and simulating attacks to see how it responds.
5. Deployment
During deployment, ensure that all security configurations are properly set, including firewall rules, API keys, and database security policies. Regularly review these configurations to ensure compliance with security policies.
6. Maintenance
Post-deployment, continuous monitoring of the application’s performance and security is necessary. Patching vulnerabilities and performing regular security assessments (including penetration testing) will help to keep the application secure as new threats emerge.
Common Application Security Threats
1. SQL Injection
SQL injection is one of the most well-known web vulnerabilities, where an attacker manipulates an SQL query by injecting malicious SQL code. This can lead to unauthorized data access, data manipulation, or even full control of the database.
Prevention:
- Use parameterized queries and prepared statements.
- Implement ORMs (Object-Relational Mappers) which abstract the database query layer and reduce the risk of SQL injection.
- Validate and sanitize all user inputs.
2. Cross-Site Scripting (XSS)
XSS vulnerabilities allow attackers to inject malicious scripts into web pages viewed by other users. This can lead to data theft, session hijacking, or defacing web pages.
Types of XSS:
- Stored XSS: The malicious script is permanently stored on the server (e.g., in a database).
- Reflected XSS: The script is immediately returned to the user without being stored.
- DOM-based XSS: The attack is based on the manipulation of the Document Object Model (DOM) in the user’s browser.
Prevention:
- Escape user inputs before displaying them on a page.
- Implement CSP to prevent unauthorized scripts from being executed.
- Use HTTPOnly cookies to protect session data from JavaScript.
3. Cross-Site Request Forgery (CSRF)
CSRF attacks trick a user into performing an unintended action on a web application in which they are authenticated, such as transferring funds or changing account settings.
Prevention:
- Use anti-CSRF tokens: Unique tokens included in every form submission that are validated by the server.
- SameSite cookies: Restrict how cookies are sent across sites to prevent cross-site forgery attacks.
Emerging Trends in Application Security
1. DevSecOps
DevSecOps is the integration of security into the DevOps model, emphasizing that security is a shared responsibility. This means automating security testing in the CI/CD pipeline, conducting code reviews for security, and continuously monitoring applications for vulnerabilities.
Key tools:
- Static Analysis Security Testing (SAST) tools that examine code for security flaws.
- Dynamic Analysis Security Testing (DAST) tools that simulate attacks against a live application.
2. Serverless Security
Serverless architectures, such as AWS Lambda and Azure Functions, introduce new security challenges. Since these environments abstract away the infrastructure, developers must focus on securing the application code itself, securing API endpoints, and implementing proper identity and access management (IAM).
Best practices:
- Ensure function isolation by limiting access to only necessary resources.
- Monitor and audit serverless function execution logs to detect unusual behavior.
Regulatory Compliance and Application Security
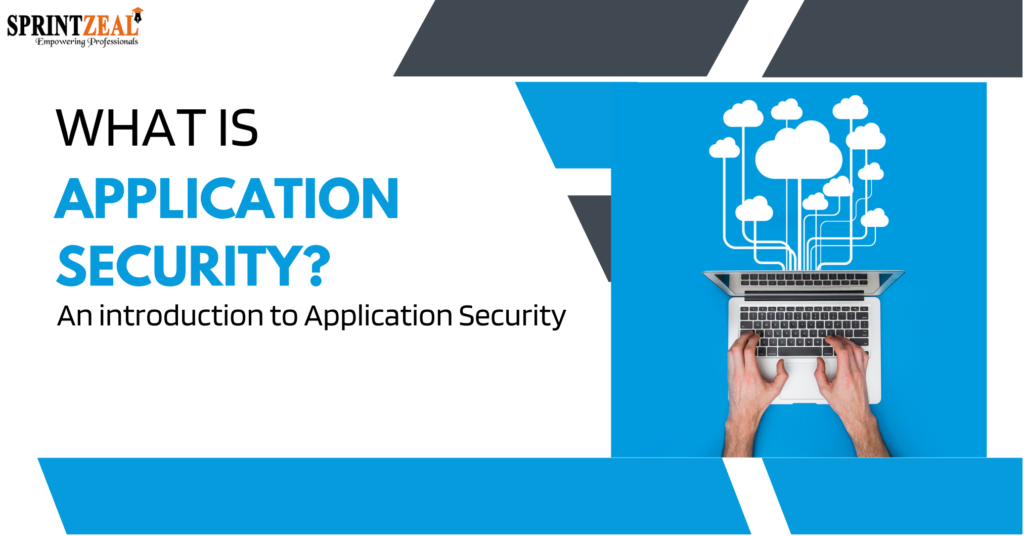
1. GDPR (General Data Protection Regulation)
GDPR is a European Union regulation that governs the handling and protection of personal data. Organizations that handle the personal data of EU citizens must comply with strict privacy standards, including:
- Data Minimization: Only collecting the necessary data for the intended purpose.
- Data Subject Rights: Allowing users to request access to their data, request correction, and demand deletion (right to be forgotten).
2. HIPAA (Health Insurance Portability and Accountability Act)
HIPAA regulates how healthcare organizations in the United States handle patient data. Compliance requires:
- Access Control: Ensuring that only authorized personnel can access patient records.
- Audit Controls: Keeping detailed logs of who accessed or modified health records.
Conclusion
In today’s interconnected digital world, application security is a critical discipline that requires continuous effort and attention. Security must be integrated into every phase of the software development lifecycle, from the initial design to post-deployment maintenance. By adopting a proactive approach to security, leveraging modern tools and techniques, and staying abreast of evolving threats, organizations can better protect their applications, data, and users.
Application security is a constantly evolving field, and developers must be prepared to adapt their strategies as new attack vectors and vulnerabilities are discovered. Whether you’re developing mobile apps, web applications, or serverless functions, a strong security foundation is essential to mitigate risks and ensure the long-term success of your software.
Different types of application security features include authentication, authorization, encryption, logging, and application security testing. Developers can also code applications to reduce security vulnerabilities.
- Authentication: When software developers build procedures into an application to ensure that only authorized users gain access to it. Authentication procedures ensure that a user is who they say they are. This can be accomplished by requiring the user to provide a user name and password when logging in to an application. Multi-factor authentication requires more than one form of authentication—the factors might include something you know (a password), something you have (a mobile device), and something you are (a thumb print or facial recognition).
Authorization: After a user has been authenticated, the user may be authorized to access and use the application. The system can validate that a user has permission to access the application by comparing the user’s identity with a list of authorized users. Authentication must happen before authorization so that the application matches only validated user credentials to the authorized user list.
Encryption: After a user has been authenticated and is using the application, other security measures can protect sensitive data from being seen or even used by a cybercriminal. In cloud-based applications, where traffic containing sensitive data travels between the end user and the cloud, that traffic can be encrypted to keep the data safe.
Logging: If there is a security breach in an application, logging can help identify who got access to the data and how. Application log files provide a time-stamped record of which aspects of the application were accessed and by whom.
Application security testing: A necessary process to ensure that all of these security controls work properly.
8j96wt